4. Manipulating Data with Perl (3)
Then let's change the variable names to something more meaningful. A variable in programming is like a box to store things. You can put numbers and characters in them. Much like x and y represent things in algebra!
Variables in perl are marked with a dollar sign $ in front of them. So the $input1 and $input2 are variables.
Now, replace $input1 with $number1. Then, replace $input2 with $number2. This is not really an important step but we do it nonetheless because it's a good programming habit to give your variables meaningful names that pertain to their use.
to
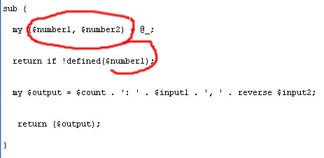
---------------------------
Now change the statement after my output="..". to "$number1 + $number2;"
The final code should look like this:
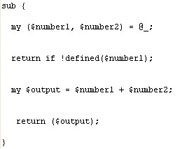
To go through the program step by step, here is what it is doing:
- the "sub" means "here is a subroutine". These can be thought of like functions in C or methods in Java.
- the line "my ($number1, $number2) = @_;" tells the subroutine to accept input and save them to the variables $number1 and $number2.
- the third line "return if !defined ($number1);" is saying "if the variable $number1 is invalid, stop right here and get out of this subroutine.
- the fourth line just tells the subroutine to add $number1 and $number 2 together, then save the result into the variable $output.
- the fifth and final line says to return the result ($output). Think of this as the program reporting back the result to you.
***if it didn't work, the user input letters will be colored red "invalid user input"***
If your program is not working, here are some things to check to make sure your perl coding is right:
- make sure all the $input1 and $input2 have been replaced with $number1 and
$number2 respectively.
- make sure all the $count variables have been removed
- make sure that there is a ! before the "define" word in the first return statement. <link to final picture for comparison>
- make sure there is a ; sign after every statement. in Programming, every letter counts!
If you are interested in learning more Perl, there are many websites on the internet to help you get started: http://www.google.com/search?hl=en&ie=UTF-8&q=perl+tutorial
Next, we shall hook up the rules to the perl program.

|